Anyways, the main problem is that the sprite's tiles do not upload to the VDP VRAM and the 1st VDP sprite list entry does not update for the sprite, according to Gen's VDP debug screen. The eagle does not appear at all. My sprite is supposed to have only 1 animation with 1 frame; however, I added a dummy frame to the spritesheet just in case an actual array of frames is needed for the SpriteDefinition struct. I noticed in the Sonic example, no VDP_ methods are used to upload the sprite's tiles, so I am assuming that the SPR_initSprite() method actually uploads the tiles to the VDP VRAM's offset for the sprite engine tile cache.
I start my FMV code by loading a background image, initializing the sprite engine to 15 tiles for the eagle, and verifying that the Sprite Engine does indeed init (it does, because "PASS!" is displayed on the screen). I then initialize the sprite's properties using PAL2 as the palette, set PAL2's contents to its palette, and then set the sprite's animation and frame to 0,0. Lastly, I move the Eagle across the screen, and display the foreground text image afterwards.
To show what the FMV is supposed to do but doesn't:
Old BEX FMV
New SGDK FMV (bugged)
Relevant and abridged code:
FMV.c:
Code: Select all
//Holds EagleSoft Ltd and Title FMV sequences
#include "../inc/fmv.h"
//EagleSoft Ltd FMV Sequence
void FMV()
{
//Variables
u16 x=0x80; //x value of Eagle Sprite
const u16 y=0xF0; //y value of Eagle Sprite
u16 offset; //Tile offset for Eagle tiles
Sprite sprites[1]; //Sprite struct
VDP_clearPlan(APLAN,0); //Clear VDP Plans A & B
VDP_clearPlan(BPLAN,0);
SYS_disableInts(); //Disable interrupts
VDP_setScreenWidth320(); //320x224 VDP mode
echo_play_sfx(SFX_33); //Play EagleSoft Ltd sfx song
VDP_setPaletteColors(0, palette_black, 64); //reset all palette to black
DrawBG(18); //Draw EagleSoft Ltd BG (Plane A)
SYS_enableInts(); //Enable interrupts
SPR_init(15);
if (SPR_isInitialized()==_FALSE)
{
VDP_setTextPalette(PAL0);
VDP_drawText("FAIL!",1,1);
}
else
{
VDP_setTextPalette(PAL0);
VDP_drawText("PASS!",1,1);
}
//Allocate 15 tiles for Eagle Sprite
offset=TILE_USERINDEX+(FMV_BG.tileset->numTile)+(FMV_Text.tileset->numTile); //Get tile offset for Eagle
SPR_initSprite(&sprites[0], &SPR_Eagle, x, y, TILE_ATTR(PAL2,TRUE,FALSE,FALSE)); //Init the Eagle Sprite
SPR_update(sprites, 1); //Update it
//VDP_setSprite(0, x, y, SPRITE_SIZE(4,3), TILE_ATTR_FULL(PAL1,1,0,0,offset), 0); //Setup the sprite
VDP_setPalette(PAL2, SPR_Eagle.palette); //Init palette PAL2 to Eagle palette
SPR_setAnimAndFrame(&sprites[0], 0,0); //Set to 0th animation
//VDP_loadTileData(SPR_Eagle, Eagle_voff, 12, 0); //Get Sprite Tile Data
//Move the Eagle from left to right on screen
for (x=0x80;x<=0x1C0;x+=1)
{
SPR_setPosition(&sprites[0],x,y); //Set new position
SPR_update(sprites, 1); //Update it
VDP_waitVSync(); //Sync
}
DrawBG(19); //Draw plane B of EagleSoft Ltd (text/logo)
waitMs(1000); //Wait 1s
VDP_fadeOutAll(1000,0); //Fade in 1s
SPR_end; //Kill sprite engine
}
Code: Select all
#include <Genesis.h>
#include <timer.h>
#include "../res/Gfx/Planes.h"
#include "../res/Gfx/Sprites.h"
#include <sprite_eng.h>
#include "../inc/main.h"
#include <vdp_pal.h>
void FMV();
void Title();
Code: Select all
//Gets 4x3 tile-sized sprites from a 8x3 tile-sized spritesheet (therefore, 2 sprites)
SPRITE SPR_Eagle "FMV\Eagle.png" 4 3 0 5
Code: Select all
#ifndef _SPRITES_H_
#define _SPRITES_H_
extern const SpriteDefinition SPR_Eagle;
#endif // _SPRITES_H_
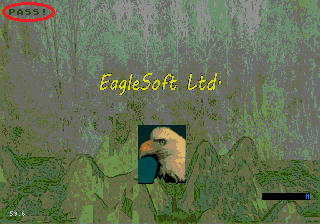
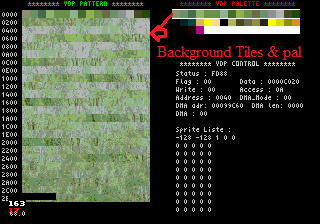
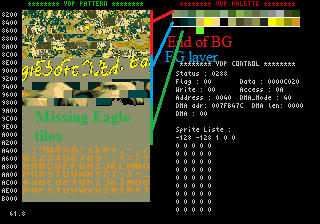
...and the current ROM source
What am I doing wrong? I really need to learn how to fix this, and deal with the sprite engine in order to fix the FMV, and, more importantly, begin working on puck and paddle movement and collisions.
Thanks in advanced!